The smallest number expressible as the sum of a prime square, prime cube, and prime fourth power is 28. In fact, there are exactly four numbers below fifty that can be expressed in such a way:
28 = 22 + 23 + 24
33 = 32 + 23 + 24
49 = 52 + 23 + 24
47 = 22 + 33 + 24
How many numbers below fifty million can be expressed as the sum of a prime square, prime cube, and prime fourth power?
Here is my solution in GoLang; it is a little more verbose than necessary but the extra checking of the upper limit of 50,000,000 and breaking loop logic. as well as caching products, expedited time to execute.
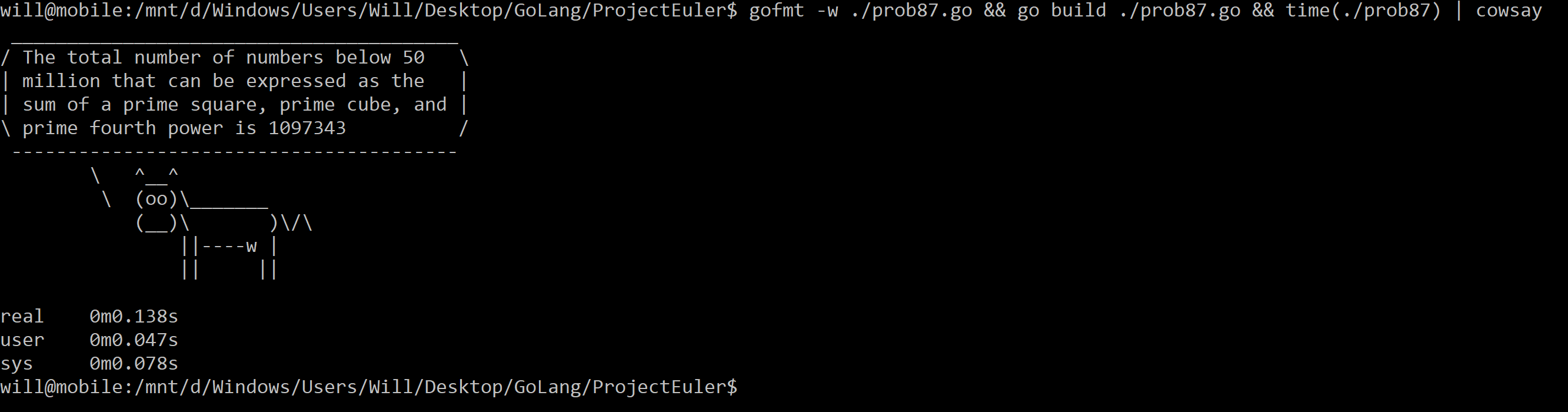
package main
import (
"fmt"
)
func main() {
primeList := sieveOfErastothenes(7071) // Sqrt of 50 million
numberFound := make([]bool, 50000000) // It's over 9 thousand!
count := 0 // Less than 9 thousand
primeListLength := len(primeList)
for i := 0; i < primeListLength; i++ {
// calculate to prevent redundant calculations
ipow := primeList[i] * primeList[i]
// 2^3 + 2^4 = 8 + 16 = 24, i is too high
if (ipow + 24) > 500000000 {
break
}
for j := 0; j < primeListLength; j++ {
jpow := primeList[j] * primeList[j] * primeList[j]
if (ipow + jpow) > 50000000 {
break
}
for k := 0; k < primeListLength; k++ {
kpow := primeList[k] * primeList[k] * primeList[k] * primeList[k]
l := ipow + jpow + kpow
if l < 50000000 {
// If false, number hasn't been marked
if !numberFound[l] {
// mark and increment counter
numberFound[l] = true
count++
}
} else {
break
}
}
}
}
fmt.Printf("The total number of numbers below 50 million that can be expressed as the sum of a prime square, prime cube, and prime fourth power is %d", count)
}
func sieveOfErastothenes(maxVal int) []int {
sieve := make([]bool, maxVal)
primeList := make([]int, 0)
primeList = append(primeList, 2)
for i := 3; i < maxVal; i += 2 {
if sieve[i] == false {
primeList = append(primeList, i)
}
for j := i * 2; j < maxVal; j += i {
sieve[j] = true
}
}
return primeList
}